4 Differences between Next.js and Remix.run
Explore the major differences between Next.js and Remix.run in terms of routing, data loading, deployment, and styling strategies.
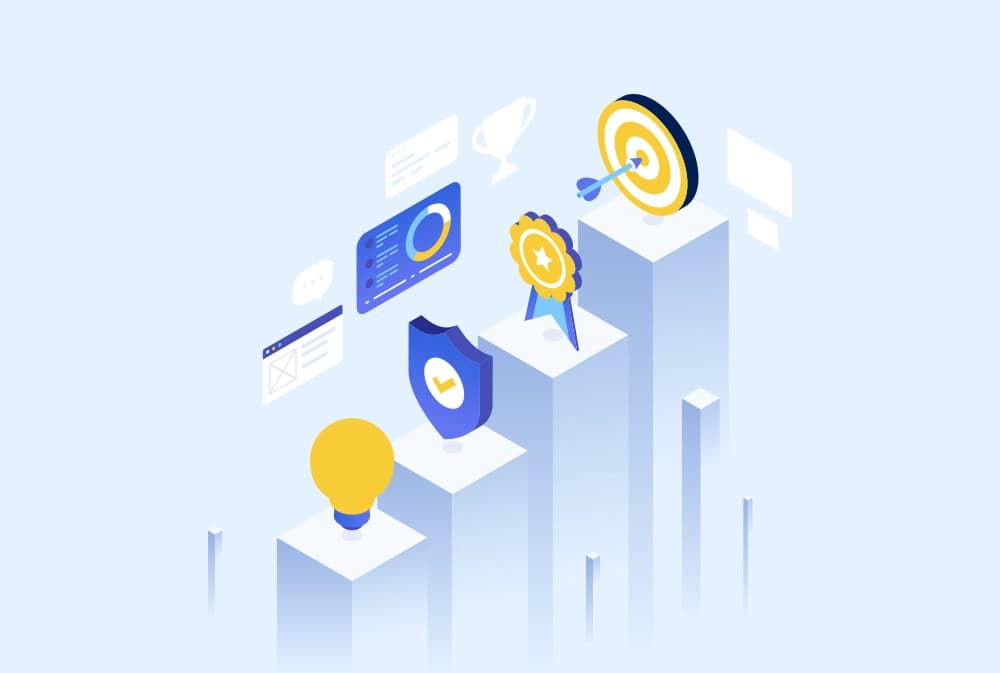
Routing
The routing system in both frameworks supports nested routing and dynamic route segments. Next.js creates routes based on the file structure inside the /pages
directory:
pages/index.js
maps to/
pages/users/index.js
maps to/users
pages/users/create.js
maps to/users/create
In Remix, the route files need to be created inside the app/routes
directory:
app/routes/index.js
maps to/
app/routes/users/index.js
maps to/users
app/routes/users/create.js
maps to/users/create
Remix routing is built on top of the React Router and allows you to utilize React Hooks, like useParams
and useNavigate
. Remix also has built-in support for nested routing with the nested layouts, while Next.js requires manual configurations.
Data Loading
Next.js offers various data loading techniques, including server-side rendering (SSR), static site generation (SSG), incremental site regeneration (ISR), and client-side rendering (CSR). You can use getServerSideProps
to load data at runtime and getStaticProps
for build time.
In contrast, Remix uses only two methods: server-side runtime rendering and client-side runtime rendering. The data is loaded using the loader
function exported from a route.
import { useLoaderData } from "remix";
export let loader = async ({ params, request }) => {
const id = params.id;
const url = new URL(request.url);
const dataLimit = url.searchParams.get("dataLimit");
return { id, dataLimit };
};
export default function FirstPage() {
let { id, dataLimit } = useLoaderData();
return (
<div>
<h1>The parameter is: {id}</h1>
<h2>The limit for url query is: {dataLimit}</h2>
</div>
);
}
Deployment
Next.js can be installed on any server that runs Node.js using next build && next start
. It has built-in support for running in serverless mode when deploying to Vercel, and it also has a Netlify adaptor for serverless deployment.
Remix was built to run on any platform, making it compatible with a wide range of server environments, including Remix App Server, Express Server, Netlify, Cloudflare Pages, Vercel, Fly.io, and Architect (AWS Lambda).
Styling
Remix provides a built-in technique for linking CSS stylesheets using link tags. Next.js comes with Styled-JSX as the default CSS-in-JS solution, allowing you to style components with encapsulated and scoped CSS.
export function links() {
return [{
rel: "stylesheet",
href: "https://test.min.css"
}];
}
In Remix, you can use the Links
tag to merge stylesheets within each nested route when rendering a component.
Conclusion
Both Next.js and Remix.run offer robust solutions for building modern web applications, but they differ significantly in routing, data loading, deployment, and styling techniques. The choice between the two often depends on specific project requirements and personal preferences.